OOP With PHP 4: Advanced OOP Concepts In PHP
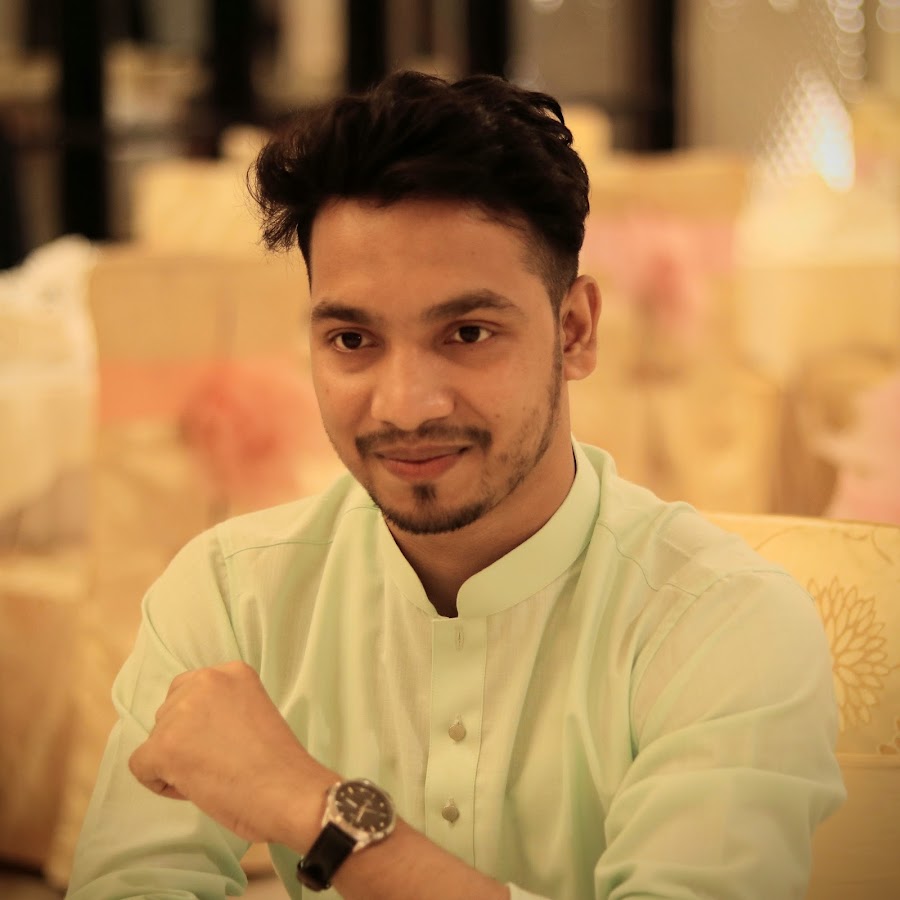
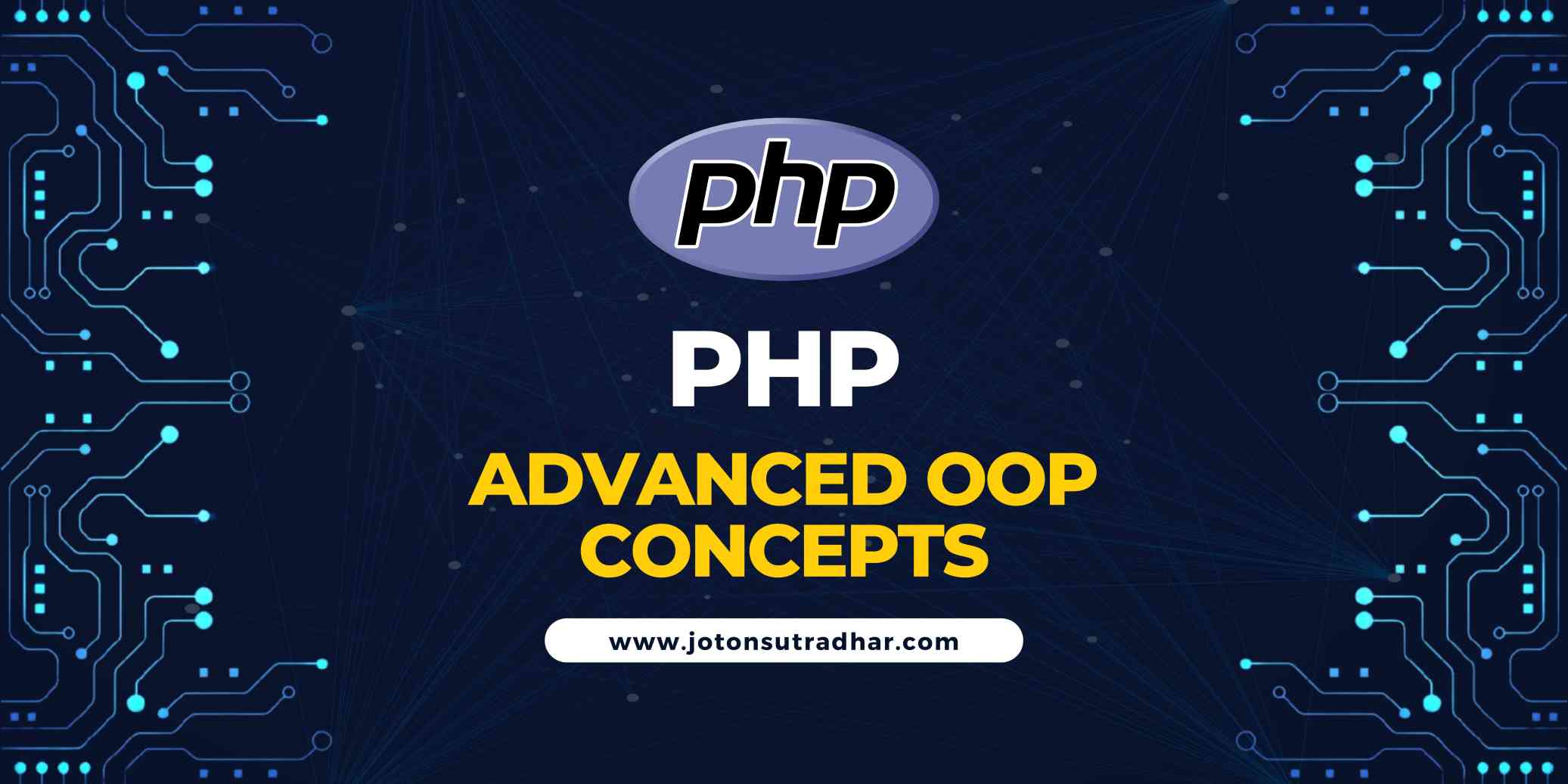
Object-Oriented Programming (OOP) in PHP provides powerful tools to build maintainable and reusable code. In this blog, we will explore three advanced OOP concepts in PHP:
-
Abstraction – Using abstract classes to define a blueprint for derived classes.
-
Interfaces – Implementing multiple behaviors in PHP classes.
-
Traits – Solving the multiple inheritance problem.
1. Abstraction in PHP: Abstract Classes & Their Usage
What is Abstraction?
Abstraction is a principle that allows defining a blueprint for a class while leaving the implementation details to the child classes. In PHP, abstract classes cannot be instantiated and must be extended by child classes.
Example:
abstract class Vehicle {
protected $speed;
public function __construct($speed) {
$this->speed = $speed;
}
// Abstract method - must be implemented in child classes
abstract public function move();
}
class Car extends Vehicle {
public function move() {
return "The car moves at a speed of {$this->speed} km/h";
}
}
$car = new Car(80);
echo $car->move();
Key Takeaways:
-
Abstract classes can have both abstract and concrete methods.
-
Child classes must implement all abstract methods.
-
Cannot instantiate an abstract class directly.
2. Interfaces in PHP: Implementing Multiple Behaviors
What is an Interface?
An interface defines a contract that classes must follow. Unlike abstract classes, an interface cannot have properties or concrete methods—only method signatures.
Example:
interface Drivable {
public function start();
public function stop();
}
interface Flyable {
public function fly();
}
class FlyingCar implements Drivable, Flyable {
public function start() {
return "The flying car starts.";
}
public function stop() {
return "The flying car stops.";
}
public function fly() {
return "The flying car is flying.";
}
}
$flyingCar = new FlyingCar();
echo $flyingCar->start() . "<br>";
echo $flyingCar->fly() . "<br>";
echo $flyingCar->stop();
Key Takeaways:
-
Interfaces enforce method implementation in classes.
-
A class can implement multiple interfaces (unlike abstract classes, which can only be extended by one class).
-
Methods in an interface must be public.
3. Traits in PHP: Solving the Multiple Inheritance Problem
What is a Trait?
Traits allow PHP to reuse methods across multiple classes without requiring inheritance. This helps solve the multiple inheritance problem, where a class needs functionality from multiple sources.
Example:
trait Logger {
public function log($message) {
echo "Logging message: $message <br>";
}
}
trait Notifier {
public function notify($user) {
echo "Notifying user: $user <br>";
}
}
class Application {
use Logger, Notifier;
public function run() {
$this->log("Application started");
$this->notify("Admin");
}
}
$app = new Application();
$app->run();
Key Takeaways:
-
Traits help avoid code duplication.
-
A class can use multiple traits.
-
Traits allow horizontal code reuse, unlike inheritance, which is hierarchical.
Conclusion
Understanding and utilizing abstraction, interfaces, and traits can significantly improve the design and maintainability of PHP applications.
-
Use Abstract Classes when creating a base blueprint with some default behavior.
-
Use Interfaces when enforcing a contract across multiple unrelated classes.
-
Use Traits when sharing methods across multiple classes without inheritance constraints.
By mastering these advanced OOP techniques, you can write more modular, reusable, and scalable PHP code!
Related Blogs
PHP is a versatile scripting language widely used for web development. To build secure and maintainable applications, it is essential to follow best practices, particularly when dealing with object-oriented programming and error handling. This article covers two crucial aspects: using the final keyword to prevent method overriding and handling exceptions effectively with try, catch, and custom exceptions.
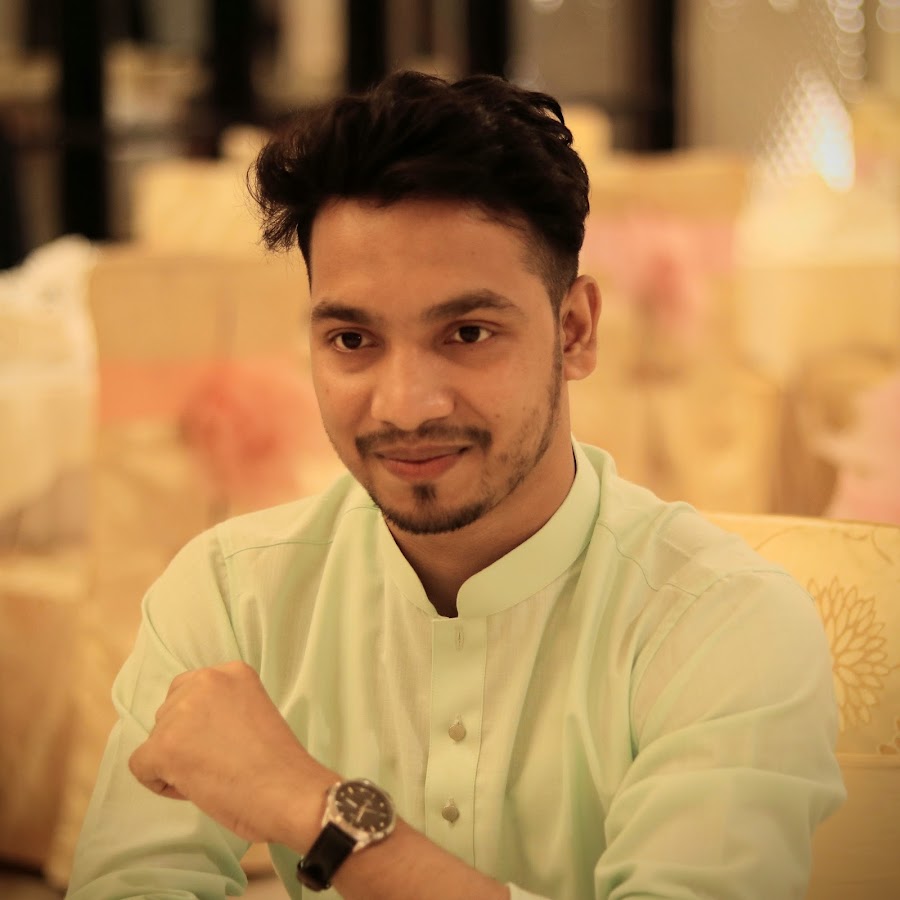
Managing large codebases efficiently is a crucial aspect of PHP development. Two key concepts that help in this regard are Namespaces and Dependency Injection.
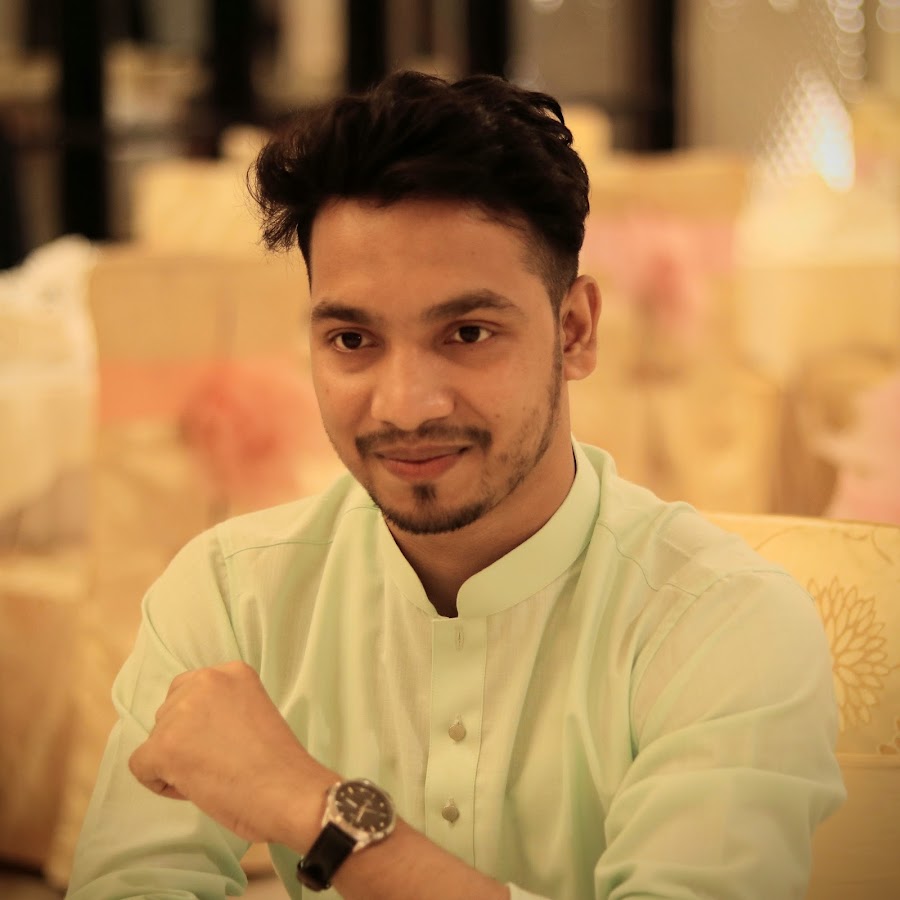
PHP provides powerful object-oriented features, including static methods and properties and magic methods, which allow developers to write cleaner and more dynamic code. In this blog post, we'll explore these concepts with practical examples.
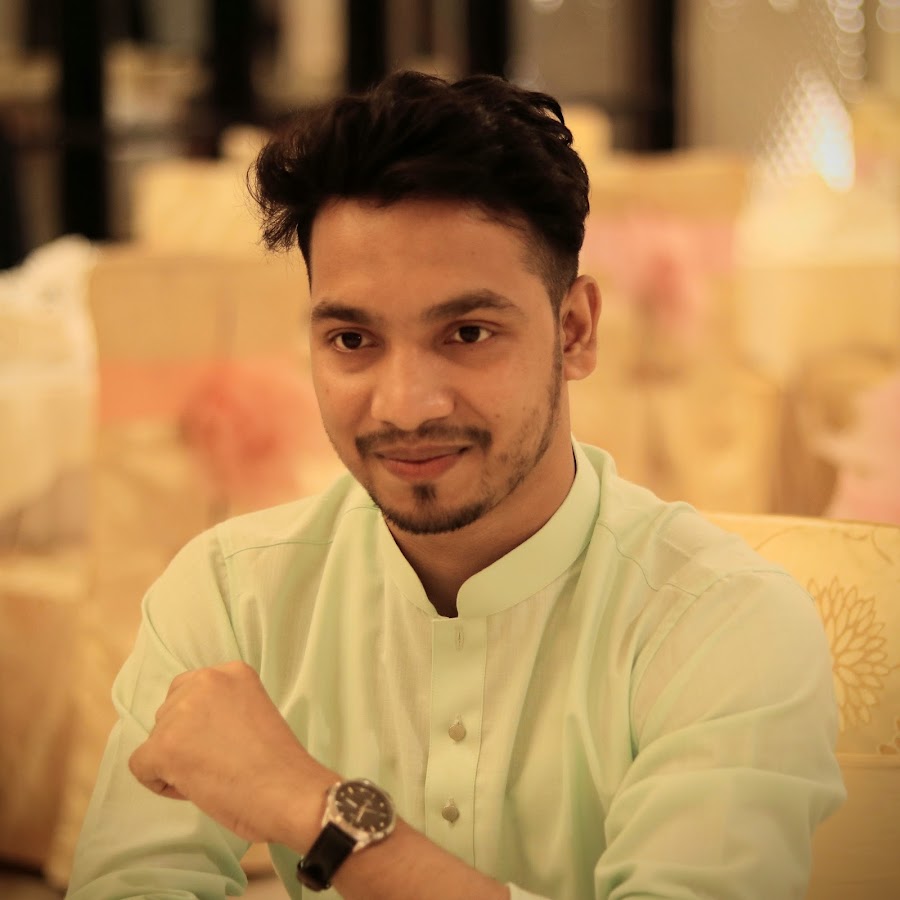