OOP With PHP 6: Namespaces And Dependency Injection
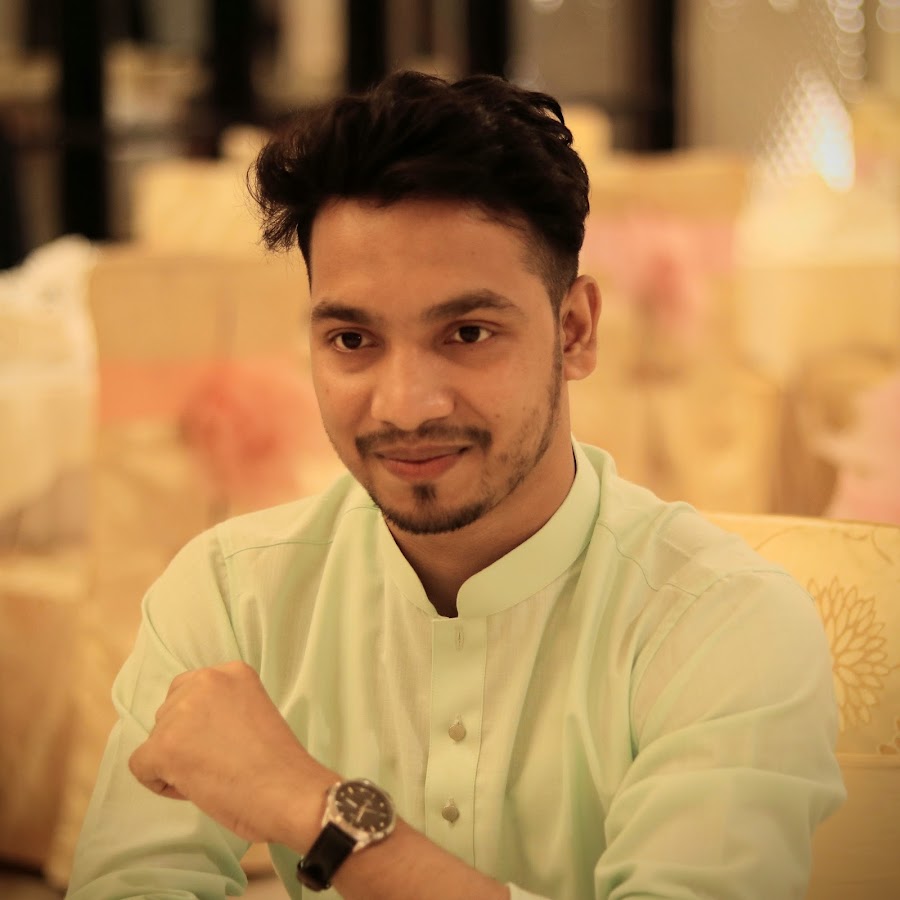
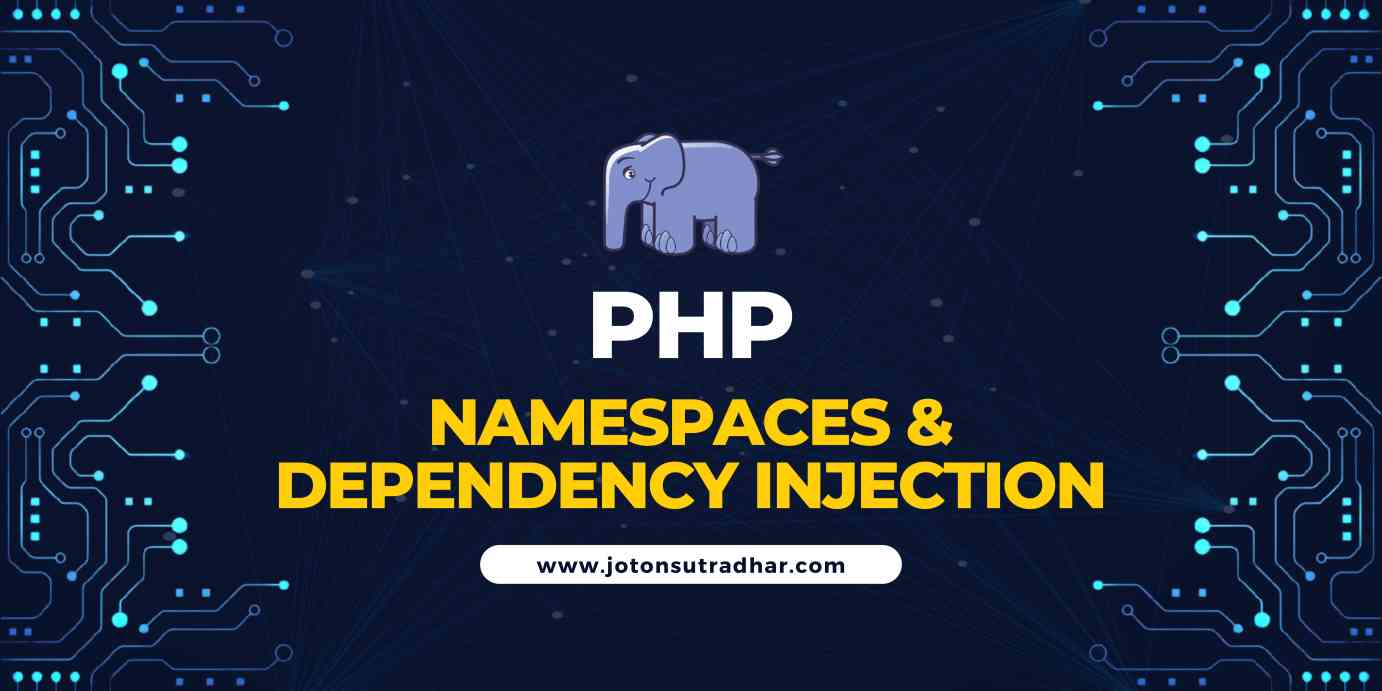
Managing large codebases efficiently is a crucial aspect of PHP development. Two key concepts that help in this regard are Namespaces and Dependency Injection.
1. Namespaces in PHP: Organizing Large Codebases
As projects grow, you may end up with multiple classes, functions, or constants that have the same name. PHP Namespaces help avoid conflicts and organize code logically.
Why Use Namespaces?
- Avoids name conflicts between classes, functions, and constants.
- Organizes code into logical groups.
- Improves maintainability and readability.
Defining a Namespace
You define a namespace at the beginning of a PHP file using the namespace
keyword.
<?php
namespace App\Models;
class User {
public function getName() {
return "John Doe";
}
}
Using Namespaces in Other Files
You can refer to namespaced classes in different ways:
1. Fully Qualified Name
$user = new \App\Models\User();
echo $user->getName();
2. Using the use
Keyword
<?php
use App\Models\User;
$user = new User();
echo $user->getName();
3. Alias with as
<?php
use App\Models\User as Customer;
$customer = new Customer();
echo $customer->getName();
Namespaces allow you to create a structured and scalable codebase.
2. Dependency Injection in PHP: Managing Dependencies & Code Maintainability
Dependency Injection (DI) is a design pattern that helps manage class dependencies, making code modular, testable, and easier to maintain.
Why Use Dependency Injection?
- Reduces tight coupling between classes.
- Enhances testability (facilitates unit testing).
- Promotes reusability and flexibility.
Without Dependency Injection (Tightly Coupled Code)
class Logger {
public function log($message) {
echo "Log: " . $message;
}
}
class UserService {
private $logger;
public function __construct() {
$this->logger = new Logger(); // Direct instantiation (tight coupling)
}
public function createUser($name) {
$this->logger->log("User {$name} created.");
}
}
$service = new UserService();
$service->createUser("John Doe");
Problem: The UserService
class is tightly coupled with the Logger
class, making it difficult to replace or mock it for testing.
With Dependency Injection (Loosely Coupled Code)
Using Dependency Injection, we inject dependencies instead of creating them inside a class.
class Logger {
public function log($message) {
echo "Log: " . $message;
}
}
class UserService {
private $logger;
// Injecting the Logger dependency via the constructor
public function __construct(Logger $logger) {
$this->logger = $logger;
}
public function createUser($name) {
$this->logger->log("User {$name} created.");
}
}
// Passing Logger as a dependency
$logger = new Logger();
$service = new UserService($logger);
$service->createUser("John Doe");
Benefits of Dependency Injection
- Flexibility – Easily switch out the
Logger
implementation. - Testability – Mock dependencies for testing.
- Scalability – Helps manage larger projects with ease.
Conclusion
- Namespaces help organize code and prevent naming conflicts.
- Dependency Injection improves code maintainability by decoupling dependencies.
By implementing these techniques, you ensure your PHP applications remain scalable, testable, and well-structured. π
Related Blogs
PHP is a versatile scripting language widely used for web development. To build secure and maintainable applications, it is essential to follow best practices, particularly when dealing with object-oriented programming and error handling. This article covers two crucial aspects: using the final keyword to prevent method overriding and handling exceptions effectively with try, catch, and custom exceptions.
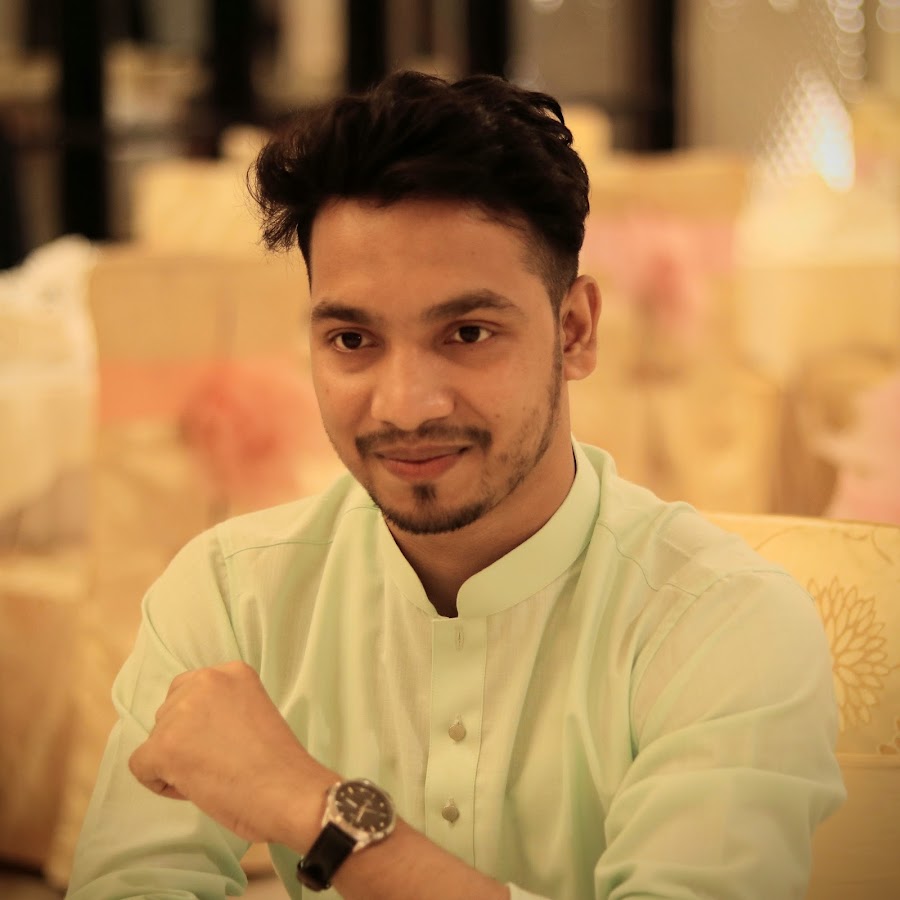
PHP provides powerful object-oriented features, including static methods and properties and magic methods, which allow developers to write cleaner and more dynamic code. In this blog post, we'll explore these concepts with practical examples.
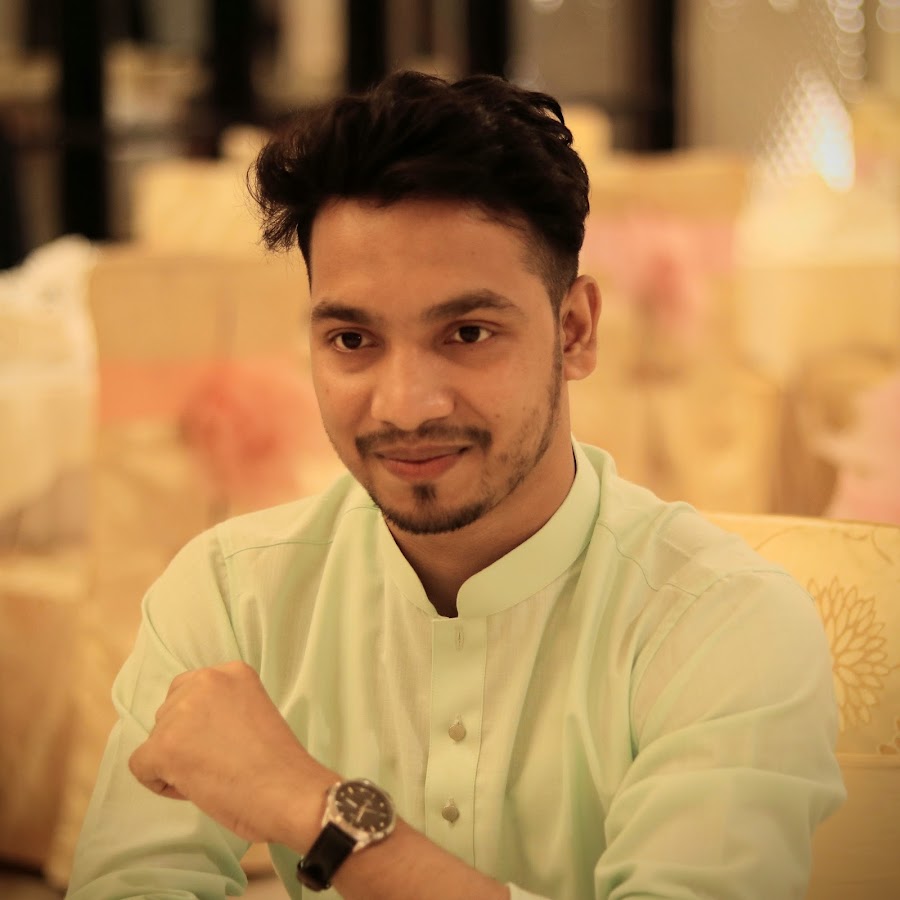
Object-Oriented Programming (OOP) in PHP provides powerful tools to build maintainable and reusable code. In this blog, we will explore three advanced OOP concepts in PHP:
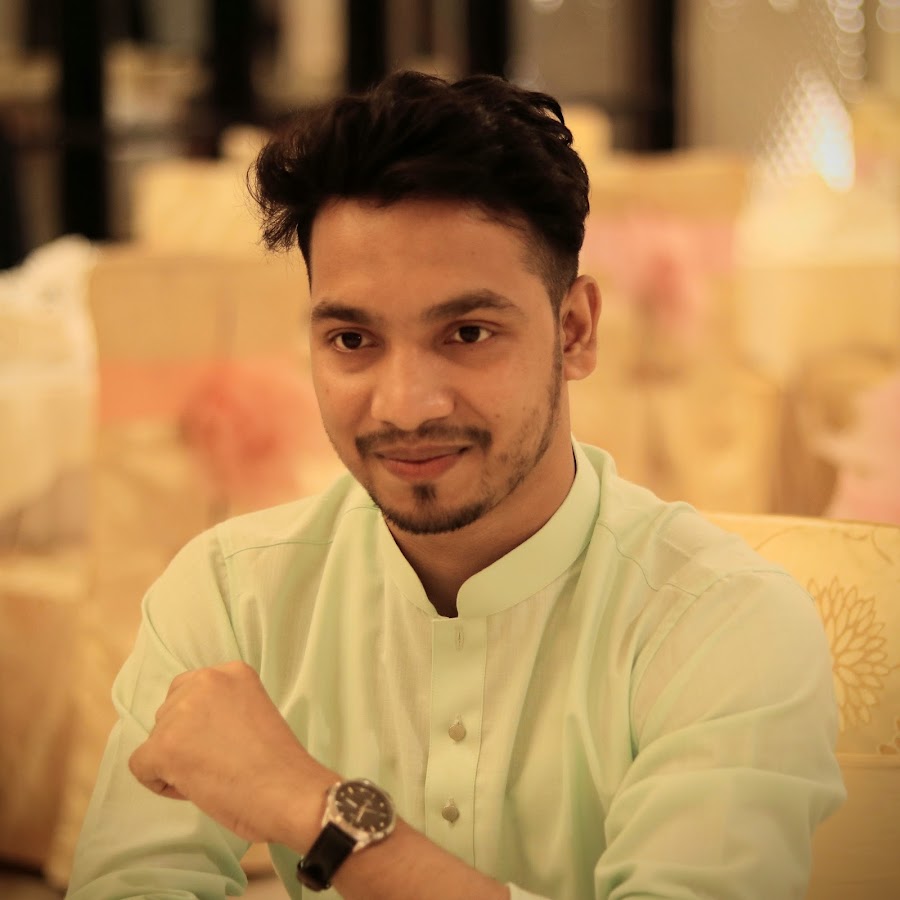