OOP With PHP 7: Best Practices & Exception Handling In PHP
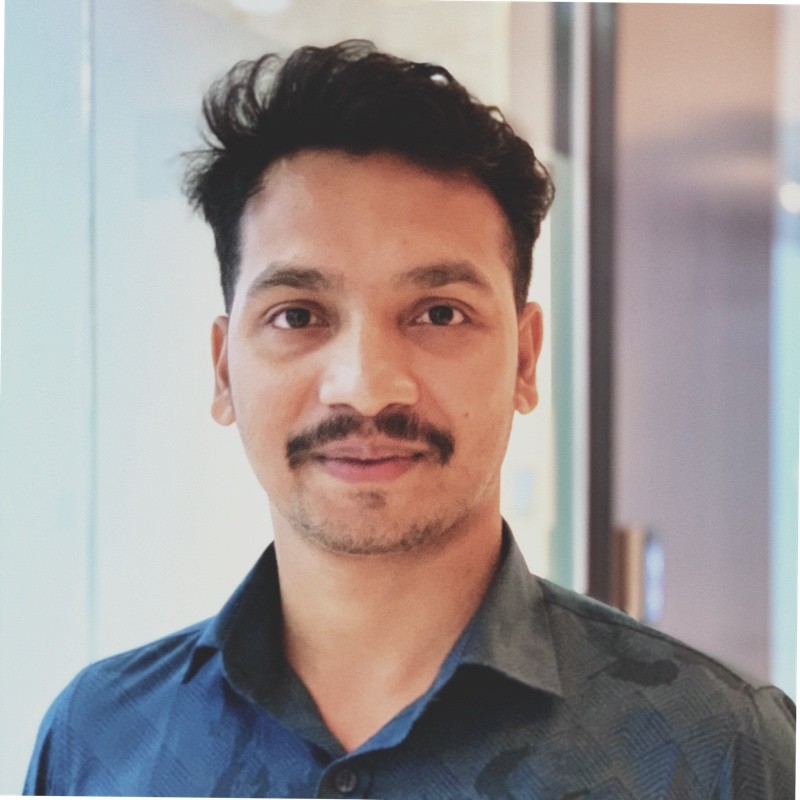
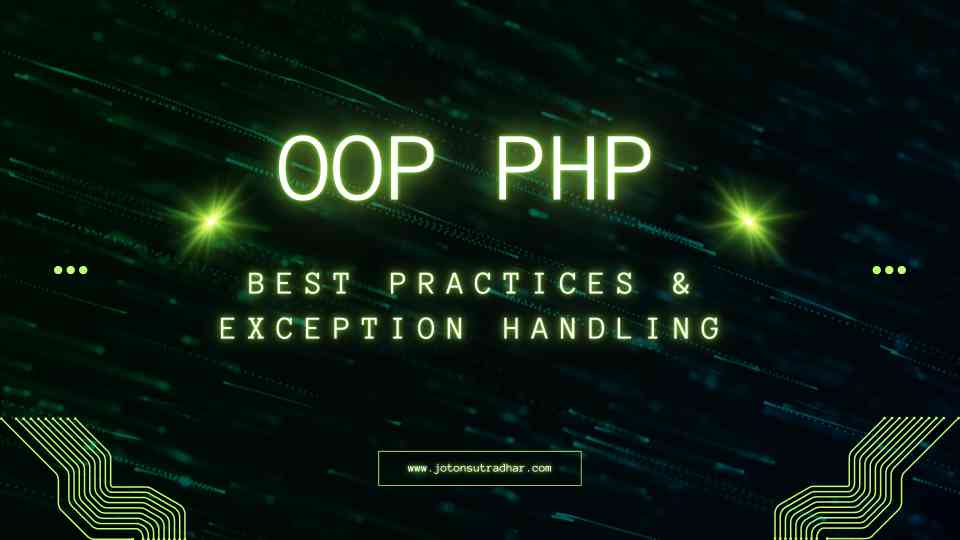
PHP is a versatile scripting language widely used for web development. To build secure and maintainable applications, it is essential to follow best practices, particularly when dealing with object-oriented programming and error handling. This article covers two crucial aspects: using the final
keyword to prevent method overriding and handling exceptions effectively with try
, catch
, and custom exceptions.
1. Using the Final Keyword in PHP to Prevent Overriding
In PHP, the final
keyword is used to prevent a class or method from being overridden by subclasses. This is particularly useful when you want to ensure that core logic remains unchanged.
Why Use final
?
-
Prevents accidental modifications to critical functionality.
-
Ensures security and stability in framework or library development.
-
Helps maintain intended behavior of a class or method.
Example: Preventing Method Overriding
class BaseClass {
final public function importantMethod() {
echo "This method cannot be overridden!";
}
}
class DerivedClass extends BaseClass {
// This will cause a fatal error
/*
public function importantMethod() {
echo "Trying to override!";
}
*/
}
Example: Preventing Class Inheritance
final class SecureClass {
public function showMessage() {
echo "This class cannot be extended!";
}
}
// This will cause a fatal error
/*
class AnotherClass extends SecureClass {
}
*/
Using final
ensures that your important methods and classes remain unchanged, avoiding unintentional breakages.
2. Exception Handling in PHP: Try, Catch, and Custom Exceptions
Exception handling in PHP helps manage errors gracefully, improving application reliability. PHP provides the try
, catch
, and throw
mechanisms for handling exceptions.
Basic Exception Handling
try {
$num = 0;
if ($num === 0) {
throw new Exception("Number cannot be zero!");
}
echo 100 / $num;
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Using Custom Exceptions
PHP allows defining custom exception classes to handle specific errors.
class CustomException extends Exception {
public function errorMessage() {
return "Custom Error: " . $this->getMessage();
}
}
try {
$age = 15;
if ($age < 18) {
throw new CustomException("Age must be 18 or older.");
}
echo "Access granted.";
} catch (CustomException $e) {
echo $e->errorMessage();
}
Best Practices for Exception Handling
-
Use Specific Exception Types - Instead of generic
Exception
, create domain-specific exceptions for clarity. -
Log Exceptions - Always log critical errors to track issues.
-
Avoid Silencing Errors - Ensure meaningful error messages are returned.
-
Use
finally
When Necessary - Release resources like database connections or file handlers.
try {
$file = fopen("test.txt", "r");
if (!$file) {
throw new Exception("File not found");
}
// Process file
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
} finally {
if ($file) {
fclose($file);
}
}
By following these best practices, you can create more reliable, maintainable PHP applications.
Subscribe for newsletter for weekly update. Happy coding! π
Related Blogs
Alright, Laravel developers, let's talk shop. We love Laravel for its elegance, convention over configuration, and how quickly it lets us build. But as our applications grow, even in a framework as opinionated as Laravel, we can fall into traps: repeating the same logic across different "service" classes, inconsistent data handling, or struggling to manage complex workflows.
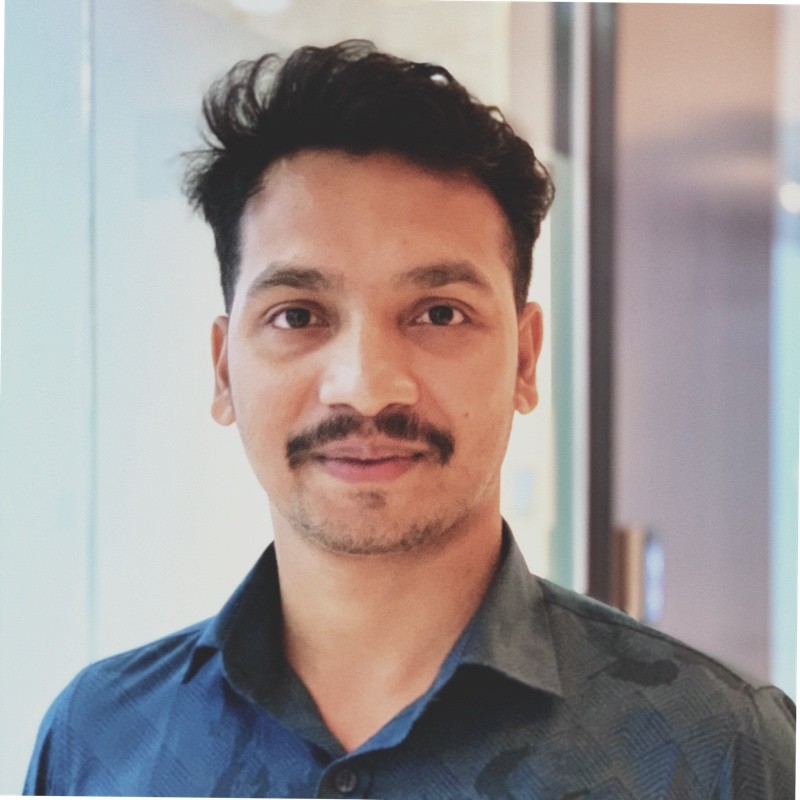
Debugging is one of the most crucial skills every developer must master, yet it's often overlooked in formal education. Whether you're a beginner writing your first "Hello World" program or a seasoned developer working on complex enterprise applications, debugging will be your constant companion throughout your coding journey.
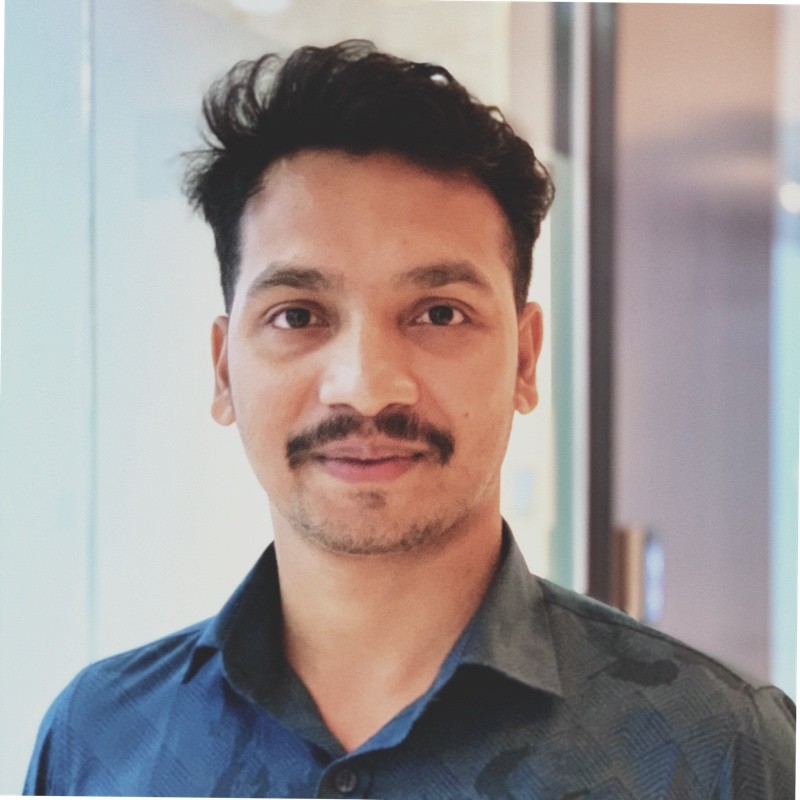
As Laravel developers, one of the critical lessons we eventually learn is: not everything should happen in real-time. Whether it's sending emails, processing images, syncing third-party data, or running analytics β pushing these resource-heavy or time-consuming tasks to the background is essential for a performant and responsive application.
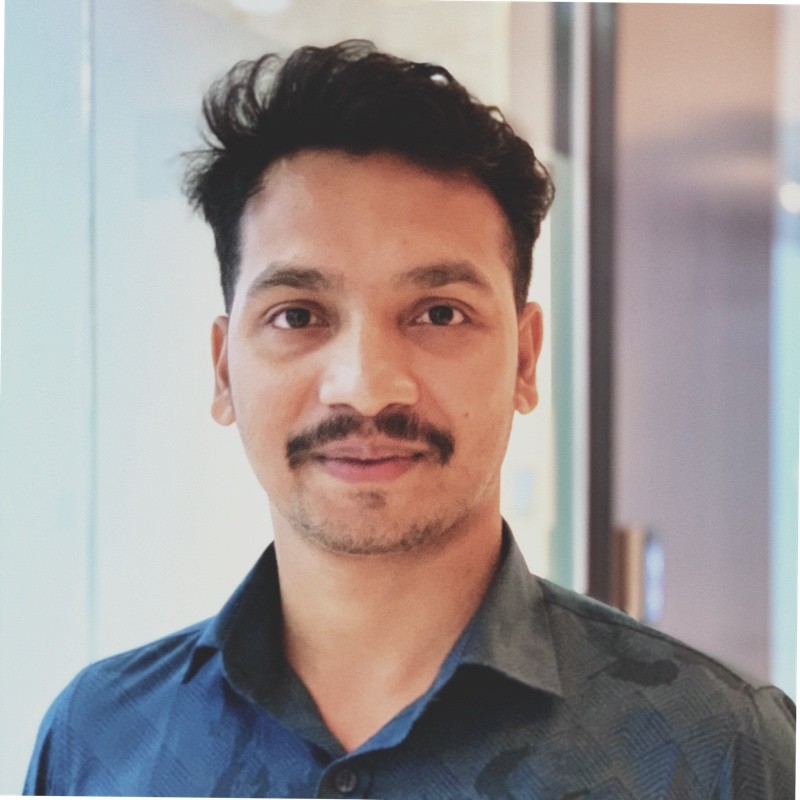