OOP With PHP 5: Static & Magic Methods In PHP
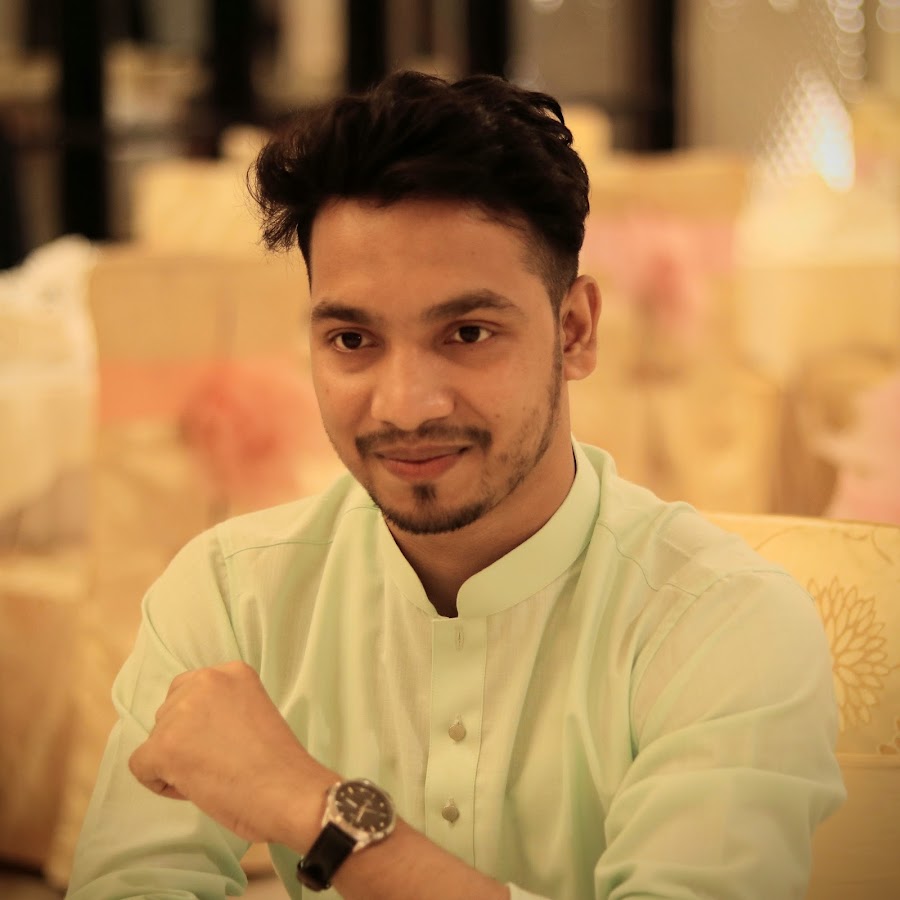
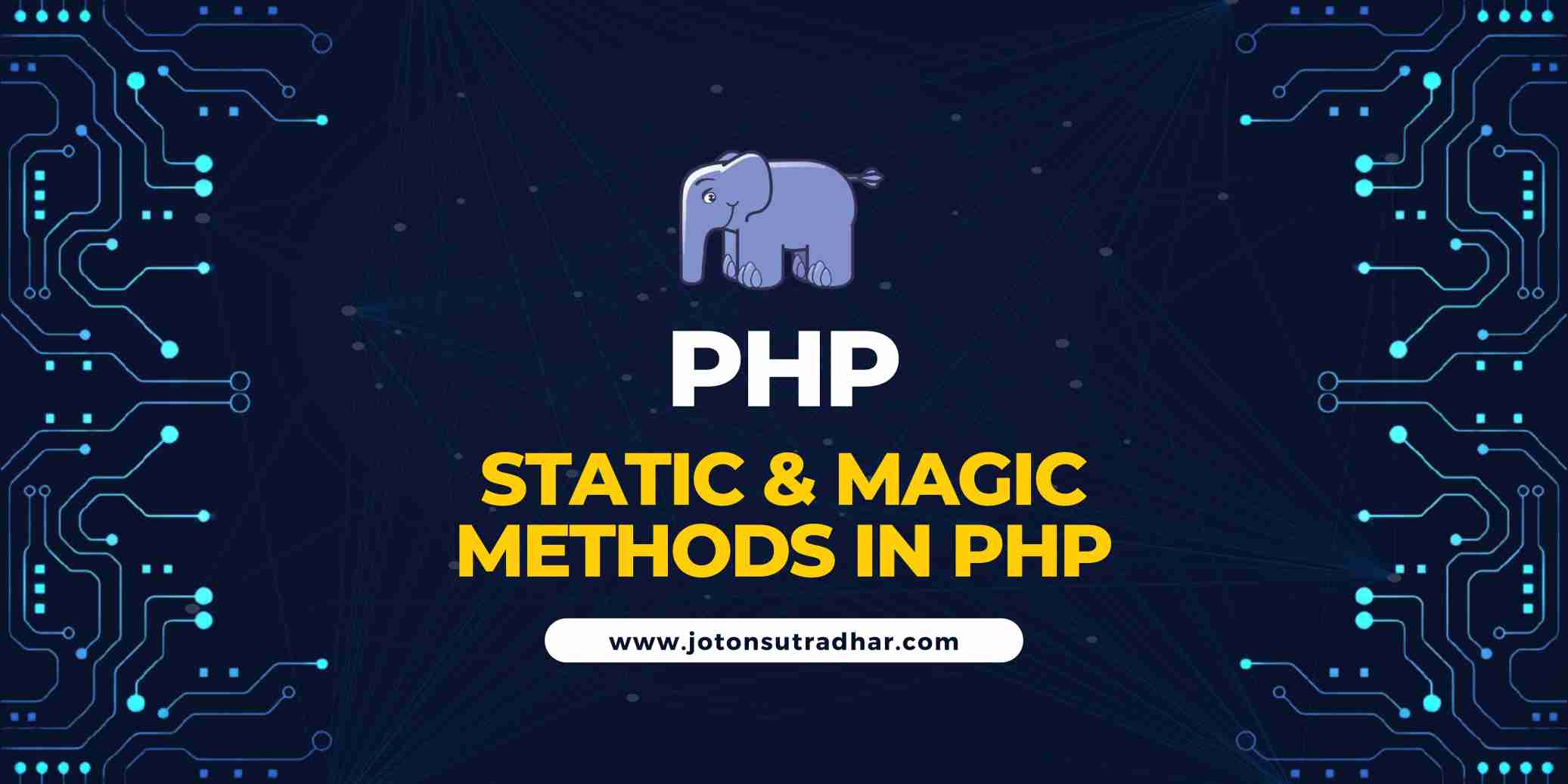
PHP provides powerful object-oriented features, including static methods and properties and magic methods, which allow developers to write cleaner and more dynamic code. In this blog post, we'll explore these concepts with practical examples.
1. Static Methods & Properties in PHP
Static methods and properties belong to a class rather than an instance of a class. They are declared using the static
keyword.
When and Why to Use Static Methods & Properties?
- When a method does not need access to instance properties.
- When working with utility/helper functions (e.g., math calculations, string manipulations).
- When maintaining a global state or shared resource.
Defining and Accessing Static Members
class MathHelper {
public static $pi = 3.14159;
public static function square($number) {
return $number * $number;
}
}
// Accessing without instantiating the class
echo MathHelper::$pi; // Output: 3.14159
echo MathHelper::square(4); // Output: 16
π‘ Note: Static properties cannot be accessed via $this
, as $this
refers to an instance.
Using self
and static
in Static Methods
class Counter {
public static $count = 0;
public static function increment() {
self::$count++; // Using 'self' to access static property
}
}
Counter::increment();
echo Counter::$count; // Output: 1
2. Understanding Magic Methods in PHP
Magic methods are predefined methods in PHP that allow you to hook into class behavior dynamically. They are prefixed with __
(double underscores).
Common Magic Methods
__construct()
– Called automatically when an object is created.__get($name)
– Invoked when accessing a non-existing property.__set($name, $value)
– Invoked when assigning a value to a non-existing property.__call($name, $arguments)
– Handles calls to undefined instance methods.__callStatic($name, $arguments)
– Handles calls to undefined static methods.__toString()
– Defines how an object is converted to a string.__invoke()
– Makes an object callable as a function.
Example of Magic Methods
1. __construct()
class User {
public $name;
public function __construct($name) {
$this->name = $name;
}
}
$user = new User("John");
echo $user->name; // Output: John
2. __get()
and __set()
class Person {
private $data = [];
public function __set($key, $value) {
$this->data[$key] = $value;
}
public function __get($key) {
return $this->data[$key] ?? "Property does not exist!";
}
}
$p = new Person();
$p->age = 30; // Calls __set
echo $p->age; // Calls __get, Output: 30
3. __call()
and __callStatic()
class Magic {
public function __call($name, $arguments) {
return "Method '$name' does not exist. Arguments: " . implode(", ", $arguments);
}
public static function __callStatic($name, $arguments) {
return "Static method '$name' does not exist.";
}
}
$obj = new Magic();
echo $obj->someMethod(1, 2, 3); // Output: Method 'someMethod' does not exist. Arguments: 1, 2, 3
echo Magic::someStaticMethod(); // Output: Static method 'someStaticMethod' does not exist.
4. __toString()
class Book {
private $title;
public function __construct($title) {
$this->title = $title;
}
public function __toString() {
return "Book Title: " . $this->title;
}
}
$book = new Book("PHP Essentials");
echo $book; // Output: Book Title: PHP Essentials
5. __invoke()
class CallableClass {
public function __invoke($message) {
return "Invoked with message: $message";
}
}
$obj = new CallableClass();
echo $obj("Hello!"); // Output: Invoked with message: Hello!
3. Method Overloading in PHP (Achieved via Magic Methods)
PHP does not support method overloading like Java or C++, but we can achieve similar functionality using __call()
and __callStatic()
.
Example: Overloading Methods Based on Argument Count
class Calculator {
public function __call($name, $arguments) {
if ($name == "add") {
if (count($arguments) == 2) {
return $arguments[0] + $arguments[1];
} elseif (count($arguments) == 3) {
return $arguments[0] + $arguments[1] + $arguments[2];
}
}
return "Invalid method call!";
}
}
$calc = new Calculator();
echo $calc->add(2, 3); // Output: 5
echo $calc->add(2, 3, 4); // Output: 9
Conclusion
- Static methods and properties are useful for utility functions and shared data across instances.
- Magic methods provide dynamic behavior to PHP classes, allowing customization of object interactions.
- Method overloading can be implemented using magic methods like
__call()
.
By leveraging these features, you can write cleaner, more flexible PHP code! π
Happy Coding π
Related Blogs
As Laravel developers, one of the critical lessons we eventually learn is: not everything should happen in real-time. Whether it's sending emails, processing images, syncing third-party data, or running analytics β pushing these resource-heavy or time-consuming tasks to the background is essential for a performant and responsive application.
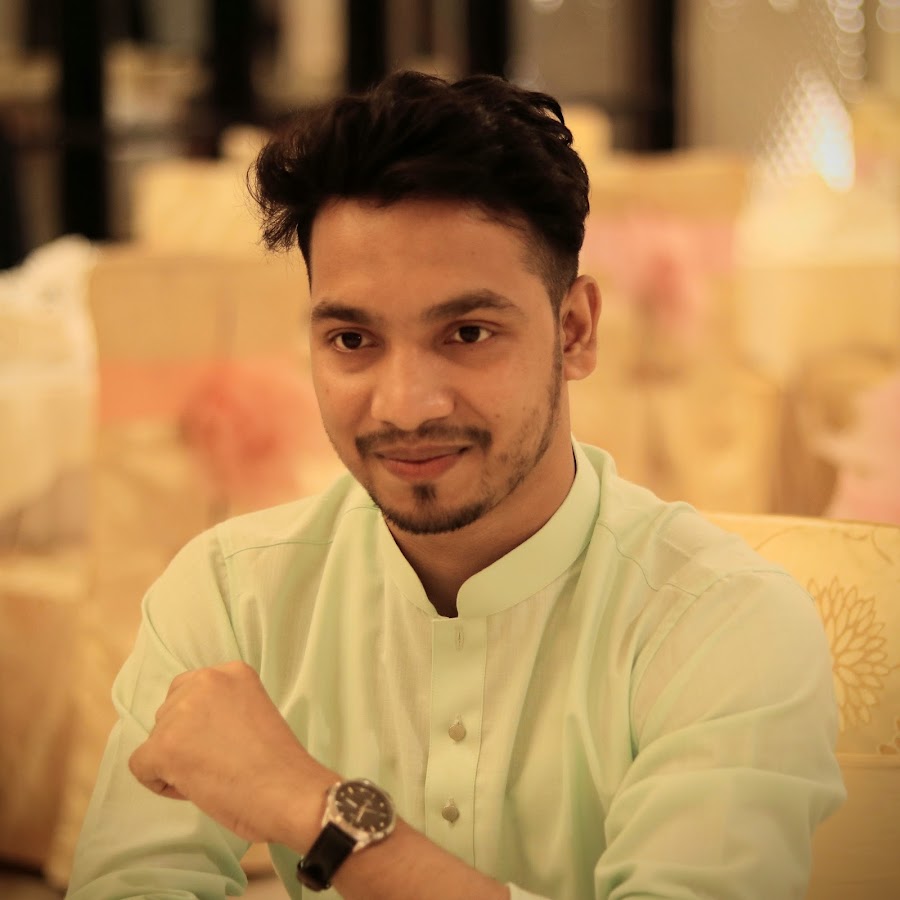
As your Laravel application grows, keeping your code organized becomes more important than ever. A bloated controller quickly becomes hard to read, test, and maintain. One of the best solutions to this problem is using the Service Pattern β a pattern that helps separate your business logic from your controllers.
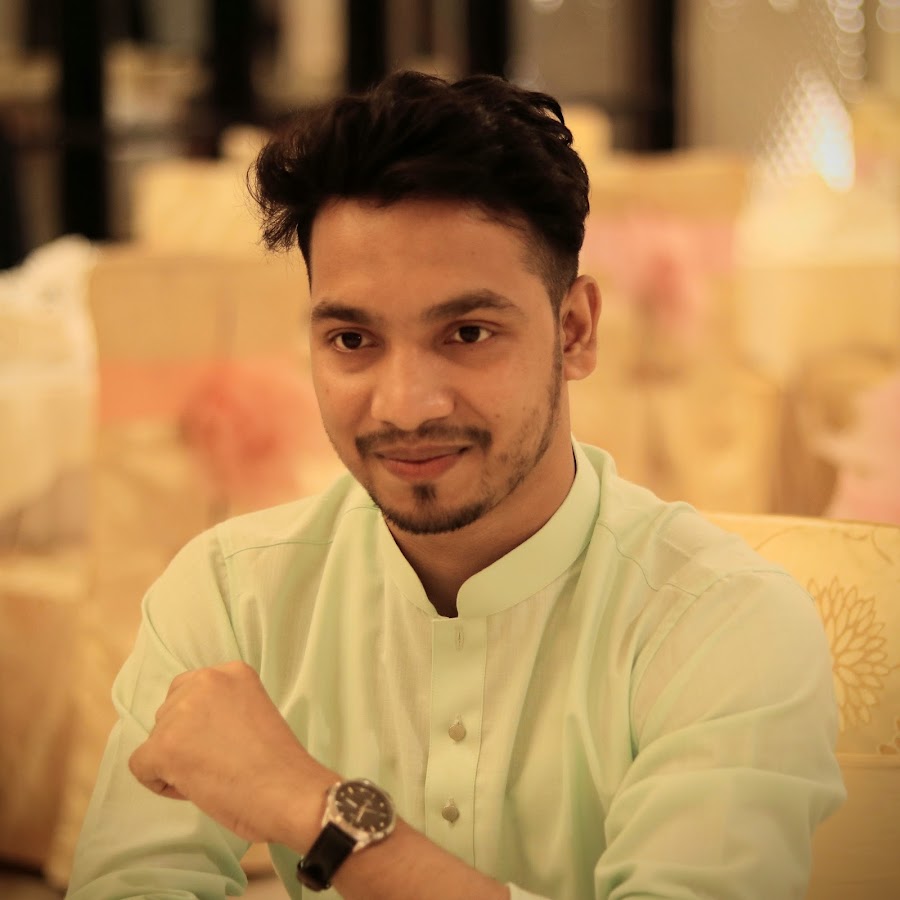
PHP is a versatile scripting language widely used for web development. To build secure and maintainable applications, it is essential to follow best practices, particularly when dealing with object-oriented programming and error handling. This article covers two crucial aspects: using the final keyword to prevent method overriding and handling exceptions effectively with try, catch, and custom exceptions.
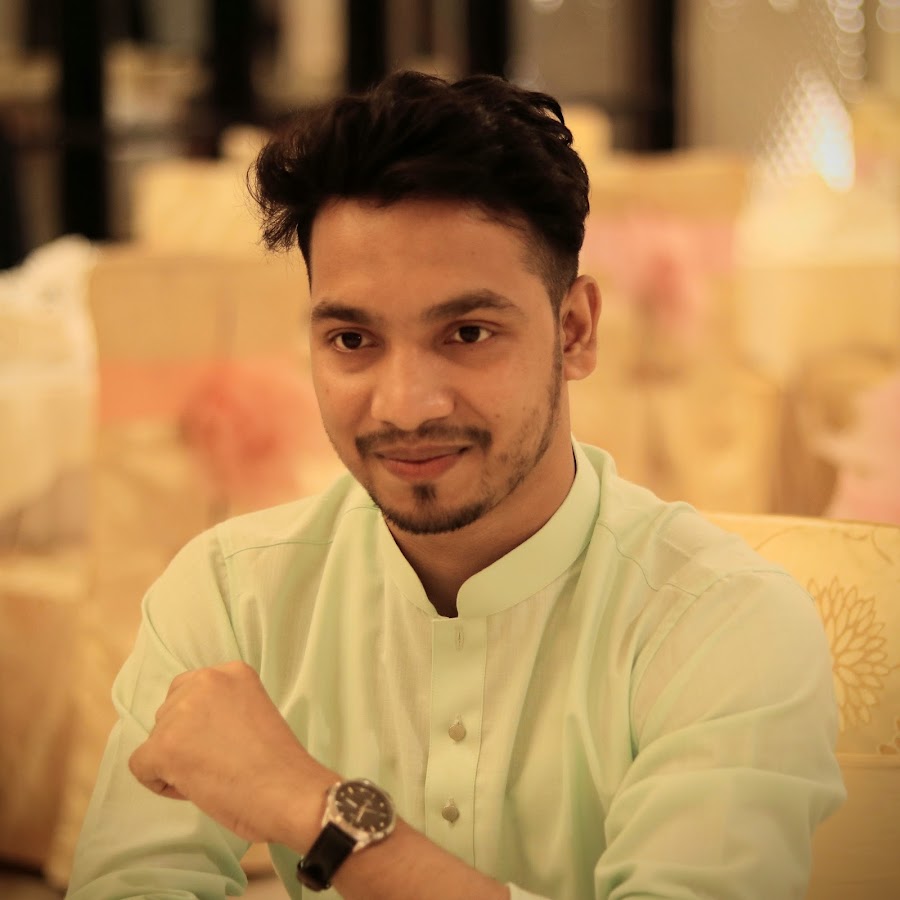